NOTE: This tutorial uses
The VB Test Harness. If you have not already created this test harness please do so first. It will make this tutorial a lot easier to follow.
This VB6 tutorial explains how to use the MsgBox function in Visual Basic. The MsgBox function displays a message in a dialog box, waits for the user to click a button, and returns an Integer indicating which button the user clicked.
Syntax:
MsgBox(prompt[, buttons] [, title] [, helpfile, context])
The MsgBox function syntax has these parts:
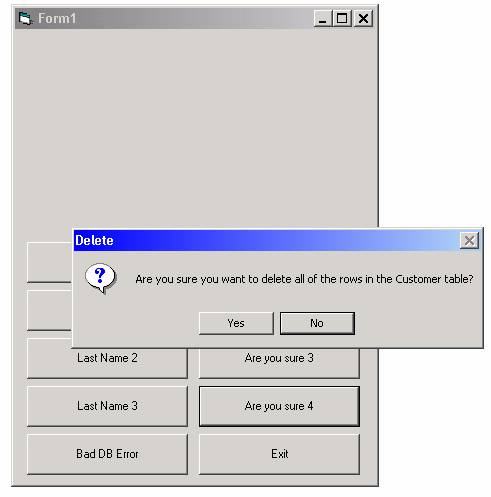
Part
Description
prompt
Required. String expression displayed as the message in the dialog box. The maximum length of prompt is approximately 1024 characters, depending on the width of the characters used.
buttons
Optional. Numeric expression that is the sum of values specifying the number and type of buttons to display, the icon style to use, the identity of the default button, and the modality of the message box. If omitted, the default value for buttons is 0 (which causes only an OK button to be displayed with no icon). The buttons argument is explained in more detail below.
title
Optional. String expression displayed in the title bar of the dialog box. If you omit title, the application name is placed in the title bar.
helpfile and context
Both optional. These arguments are only applicable when a Help file has been set up to work with the application.
The buttons argument
The first group of values (0–5) describes the number and type of buttons displayed in the dialog box; the second group (16, 32, 48, 64) describes the icon style; the third group (0, 256, 512, 768) determines which button is the default; and the fourth group (0, 4096) determines the modality of the message box. When adding numbers to create a final value for the buttons argument, use only one number from each group.
First Group - Determines which buttons to display:
Constant
Value
Description
vbOKOnly
0
Display OK button only.
vbOKCancel
1
Display OK and Cancel buttons.
vbAbortRetryIgnore
2
Display Abort, Retry, and Ignore buttons.
vbYesNoCancel
3
Display Yes, No, and Cancel buttons.
vbYesNo
4
Display Yes and No buttons.
vbRetryCancel
5
Display Retry and Cancel buttons.
Second Group - Determines which icon to display:
Constant
Value
Description
Icon
vbCritical
16
Display Critical Message icon.
vbQuestion
32
Display Warning Query (question mark) icon.
vbExclamation
48
Display Warning Message icon.
vbInformation
64
Display Information Message icon.
Third Group - Determines which button is the default:
Constant
Value
Description
vbDefaultButton1
0
First button is default.
vbDefaultButton2
256
Second button is default.
vbDefaultButton3
512
Third button is default.
vbDefaultButton4
768
Fourth button is default (applicable only if a Help button has been added).
Fourth Group Determines the modality of the message box. Note – generally, you would not need to use a constant from this group, as you would want to use the default (application modal). If you specified "system modal", you would be "hogging" Windows – i.e., if a user had another app open, like Word or Excel, they would not be able to get back to it until they responded to your app's message box.
Constant
Value
Description
vbApplicationModal
0
Application modal; the user must respond to the message box before continuing work in the current application.
vbSystemModal
4096
System modal; all applications are suspended until the user responds to the message box.
There is a fifth group of constants that can be used for the buttons argument which would only be used under special circumstances:
Constant
Value
Description
vbMsgBoxHelpButton
16384
Adds Help button to the message box
VbMsgBoxSetForeground
65536
Specifies the message box window as the foreground window
vbMsgBoxRight
524288
Text is right aligned
vbMsgBoxRtlReading
1048576
Specifies text should appear as right-to-left reading on Hebrew and Arabic systems
When you use MsgBox to with the option to display more than one button (i.e., from the first group, anything other than "vbOKOnly"), you can test which button the user clicked by comparing the return value of the Msgbox function with one of these values:
Constant
Value
Description
vbOK
1
The OK button was pressed
vbCancel
2
The Cancel button was pressed
vbAbort
3
The Abort button was pressed
vbRetry
4
The Retry button was pressed
vbIgnore
5
The Ignore button was pressed
vbYes
6
The Yes button was pressed
vbNo
7
The No button was pressed
Note: To try any of the MsgBox examples, you can simply start a new project, double-click on the form, and place the code in the Form_Load event.
There are two basic ways to use MsgBox, depending on whether or not you need to know which button the user clicked.
· If you do NOT need to test which button the user clicked (i.e., you displayed a message box with only an OK button), then you can use MsgBox as if you were calling a Sub. You can use the following syntax:
Msgbox arguments
-or-
Call MsgBox(arguments)
Examples:
o The statement
MsgBox "Hello there!"
causes the following box to be displayed
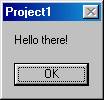
This is the simplest use of MsgBox: it uses only the required prompt argument. Since the buttons argument was omitted, the default (OK button with no icons) was used; and since the title argument was omitted, the default title (the project name) was displayed in the title bar.
o The statement
MsgBox "The Last Name field must not be blank.", _
vbExclamation, _
"Last Name"
causes the following box to be displayed:
This is how a data entry error might be displayed. Note that vbExclamation was specified for the buttons argument to specify what icon should be displayed – the fact that we did not add a value from the first group still causes only the OK button to be displayed. If you wanted to explicitly indicate that only the OK button should be displayed along with the exclamation icon, you could have coded the second argument as
vbExclamation + vbOKOnly
making the full statement read:
MsgBox "The Last Name field must not be blank.", _
vbExclamation + vbOKOnly, _
"Last Name"
Remember, for the buttons argument, you can add one value from each of the four groups.
An alternative (not recommended) is to use the hard-coded number for the buttons argument, as in:
MsgBox "The Last Name field must not be blank.", 48, "Last Name"
Note also that this example provided a value for the title argument ("Last Name"), which causes that text to be displayed in the box's title bar.
The format of the MsgBox statement used in this example could also be used for more critical errors (such as a database problem) by using the vbCritical icon. You may also want to use the name of the Sub or Function in which the error occurred for your title argument.
Example:
MsgBox "A bad database error has occurred.", _
vbCritical, _
"UpdateCustomerTable"
Result:
· If you DO need to test which button the user clicked (i.e., you displayed a message box with more than one button), then you must use MsgBox as a function, using the following syntax:
IntegerVariable = Msgbox (arguments)
One of the more common uses of MsgBox is to ask a Yes/No question of the user and perform processing based on their response, as in the following example:
Dim intResponse As Integer
intResponse = MsgBox("Are you sure you want to quit?", _
vbYesNo + vbQuestion, _
"Quit")
If intResponse = vbYes Then
End
End If
The following message box would be displayed:
After the user clicks a button, you would test the return variable (intResponse) for a value of vbYes or vbNo (6 or 7).
Note that the use of the built-in constants makes the code more readable. The statement
intResponse = MsgBox("Are you sure you want to quit?", _
vbYesNo + vbQuestion, _
"Quit")
is more readable than
intResponse = MsgBox("Are you sure you want to quit?", 36, "Quit")
and
If intResponse = vbYes Then
is more readable than
If intResponse = 6 Then
In that you can use a function anywhere a variable can be used, you could use the MsgBox function directly in an if statement without using a separate variable to hold the result ("intResponse" in this case). For example, the above example could have been coded as:
If MsgBox("Are you sure you want to quit?", _
vbYesNo + vbQuestion, _
"Quit")= vbYes Then
End
End If
Note: If desired you could place the code for this example in the cmdExit_Click event of any of the "Try It" projects.
Following is an example using the vbDefaultButton2 constant:
Dim intResponse As Integer
intResponse = MsgBox("Are you sure you want to delete all of the rows " _
& "in the Customer table?", _
vbYesNo + vbQuestion + vbDefaultButton2, _
"Delete")
If intResponse = vbYes Then
' delete the rows ...
End If
The message box displayed by this example would look like this:
he sample project for this topic contains a command button for each MsgBox example given above.